Android 5.0 推出了底部選單列 - Bottom Sheets
讓使用者可由屏幕底端滑出選單, 提供選擇或是資訊, 也可設計成跳轉至不同App的選單列.
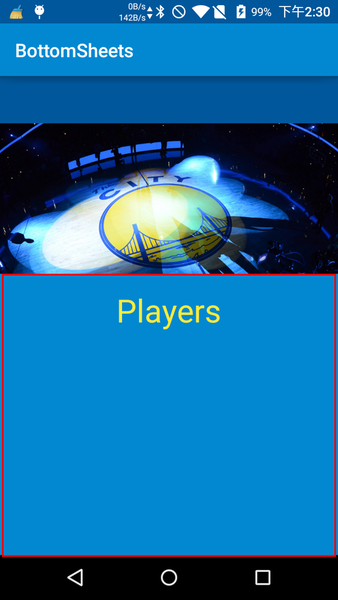
以下為設計步驟.
Step 1: 引用 Design Library
build.gradle1 2 3 4 5 6
| dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) testCompile 'junit:junit:4.12' compile 'com.android.support:appcompat-v7:23.3.0' compile 'com.android.support:design:23.3.0' }
|
appcompat-v7 與 design 版本需相同.
若使用appcompat-v7:23.3.0, 而design:23.2.0時, 無法使用.
Step 2: 編輯 Bottom Sheet 內容
在res/layout 新增 xml 檔案 : bottom_sheets_main_layout.xml, 目前先使用 TextView 當作內容
bottom_sheets_main_layout.xml1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:id="@+id/bottom_sheet" android:layout_width="match_parent" android:layout_height="300dp" android:orientation="vertical" android:padding="16dp" android:background="@color/colorPrimary" app:layout_behavior="@string/bottom_sheet_behavior">
<TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:text="Players" android:textAppearance="@style/TextAppearance.AppCompat.Display1" android:textColor="@color/colorAccent"/> </LinearLayout>
|
Step 3: 設置主畫面
在主畫面中, 使用CoordinatorLayout, 並引用Bottom Sheet內容.
activity_main.xml1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <?xml version="1.0" encoding="utf-8"?> <android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:background="@color/colorPrimaryDark">
<ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/background"/>
<include layout="@layout/bottom_sheets_main_layout" /> </android.support.design.widget.CoordinatorLayout>
|
Step 4: 設置 Bottom Sheet 行為
在MainActivity.java, 使用 BottomSheetBehavior.from 載入BottomSheet內容, 並且設定BottomSheet行為.
STATE_COLLAPSED : 必須搭配 setPeekHeight, 設定縮起後最小高度, 預設最小高度為0
STATE_EXPANDED : 將內容全部展開
STATE_HIDDEN : 全部隱藏
MainActivity.java1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| import android.support.design.widget.BottomSheetBehavior; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.LinearLayout;
public class MainActivity extends AppCompatActivity {
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
LinearLayout bottomSheetViewgroup = (LinearLayout) findViewById(R.id.bottom_sheet);
BottomSheetBehavior bottomSheetBehavior = BottomSheetBehavior.from(bottomSheetViewgroup); bottomSheetBehavior.setState(BottomSheetBehavior.STATE_COLLAPSED); bottomSheetBehavior.setPeekHeight(150); } }
|
執行結果
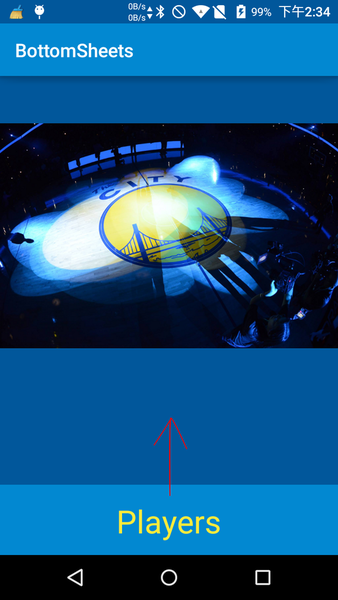
Bottom Sheet 分為兩種設計模式
- Modal Bottom Sheets :
使用時機: 與本頁無關的內容, 或是下一步的連結
使用方式: Dialog 表示 (背景會暗掉)
- Persistent Bottom Sheets
使用時機: 與本頁有關的內容
使用方式: 內文表示 (背景不會暗掉, 亮度與Bottom Sheet一致)
Persistent Bottom Sheets
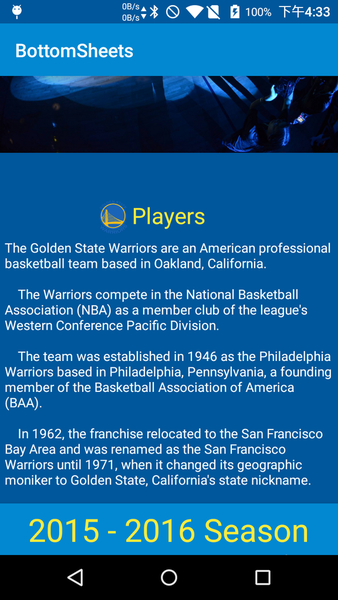
拉動後
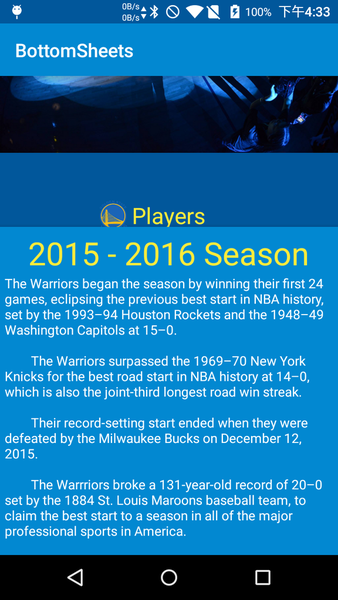
Modal Bottom Sheets
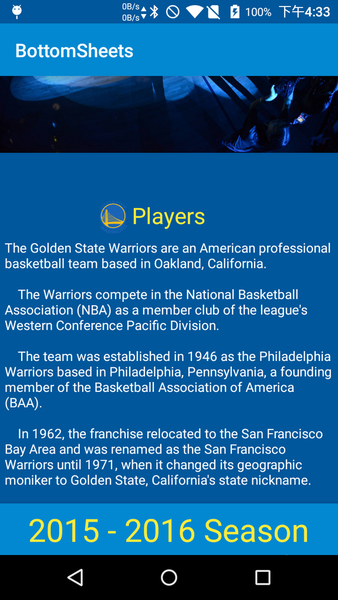
拉動後
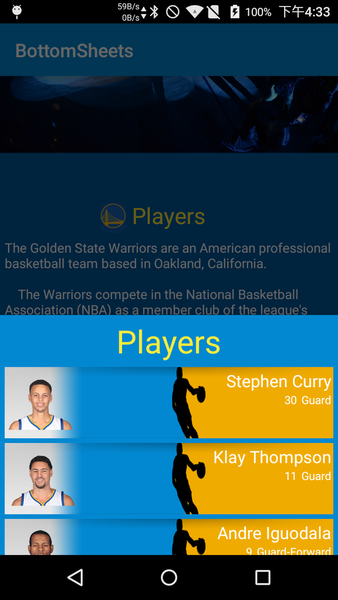
Modal Bottom Sheets 的設計請參考Android Modal Bottom Sheets