當畫面中有UIImageView且需從圖片庫中選取圖片時, 該如何實作呢?
Step 1: 設定圖片並Reference到Source Code
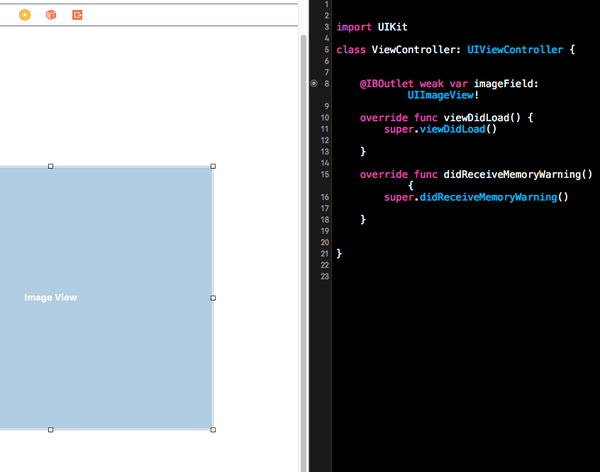
Step 2: 設定點擊圖片的處理
由於UIImageView沒有Click Event, 所以我們使用UITapGestureRecognizer來實現
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @IBOutlet weak var imageField: UIImageView! override func viewDidLoad() { super.viewDidLoad()
let tapGestureRecognizer = UITapGestureRecognizer(target:self, action:#selector(ViewController.imageClick(_:))) imageField.userInteractionEnabled = true imageField.addGestureRecognizer(tapGestureRecognizer) }
override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() }
internal func imageClick(img: AnyObject) { }
|
Step 3: 請求使用圖片庫的權限
1 2 3 4 5 6
| internal func imageClick(img: AnyObject) { if UIImagePickerController.isSourceTypeAvailable(.PhotoLibrary){ } else { print("Can't read the album!") } }
|
Step 4: 設定UIImagePickerController
使用UIImagePickerController選取圖片, 並註冊 UIImagePickerControllerDelegate & UINavigationControllerDelegate
1 2 3 4 5 6 7 8 9 10 11 12 13
| internal func imageClick(img: AnyObject) { if UIImagePickerController.isSourceTypeAvailable(.PhotoLibrary){ let picker = UIImagePickerController() picker.delegate = self picker.sourceType = UIImagePickerControllerSourceType.PhotoLibrary picker.allowsEditing = true; self.presentViewController(picker, animated: true, completion: { () -> Void in }) } else { print("Can't read the album!") } }
|
1 2 3 4 5 6 7
| extension ViewController : UIImagePickerControllerDelegate { func imagePickerController(picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [String : AnyObject]) { } }
extension ViewController : UINavigationControllerDelegate { }
|
如果應用程式只使用Landscape 的話, 會發生UIApplicationInvalidInterfaceOrientation Exception
Reason: ‘Supported orientations has no common orientation with the application, and [PUUIAlbumListViewController shouldAutorotate] is returning YES’
在iOS 的 Docutment 中提到UIUmagePickerController只支援portrait mode.
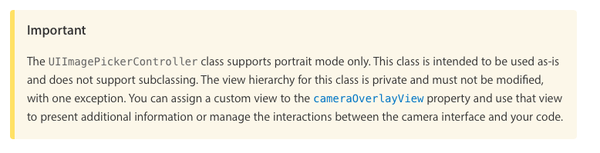
所以要將Portrait選項打勾
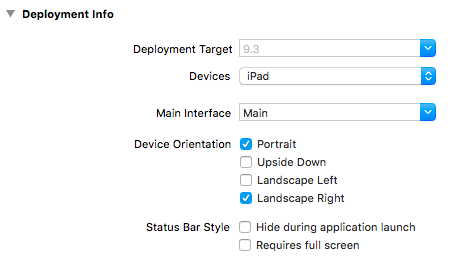
Step 5: 使用客製化UIImagePickerController, 來維持Landscape
若接受螢幕可自動切換 Landscape/Portrait時, 可跳過此步驟.
若你的應用程式為Landscape時, 可使用客製化的UIImagePickerController並覆寫以下程式碼.
- shouldAutorotate : 將自動旋轉屏幕關閉
- supportedInterfaceOrientatios
- preferredInterfaceOrientationForPresentation : 設定為Landscape
CustomImagePicker Source Code:
CustomImagePicker.swift1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| import UIKit
class CustomImagePicker: UIImagePickerController { override func viewDidLoad() { super.viewDidLoad() }
override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() }
override func shouldAutorotate() -> Bool { return false }
override func supportedInterfaceOrientations() -> UIInterfaceOrientationMask { return UIInterfaceOrientationMask(rawValue: UIInterfaceOrientationMask.LandscapeRight.rawValue); }
override func preferredInterfaceOrientationForPresentation() -> UIInterfaceOrientation { return UIInterfaceOrientation.LandscapeRight; } }
|
將程式碼改為
1 2 3 4 5 6 7 8 9 10 11 12 13
| internal func imageClick(img: AnyObject) { if UIImagePickerController.isSourceTypeAvailable(.PhotoLibrary){ let picker = CustomImagePicker() picker.delegate = self picker.sourceType = UIImagePickerControllerSourceType.PhotoLibrary picker.allowsEditing = true; self.presentViewController(picker, animated: true, completion: { () -> Void in }) } else { print("Can't read the album!") } }
|
Step 6: 設置圖片並關閉UIImagePicker
1 2 3 4 5 6 7 8
| extension ViewController : UIImagePickerControllerDelegate { func imagePickerController(picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [String : AnyObject]) {
let image = info[UIImagePickerControllerOriginalImage] as! UIImage imageField.image = image; picker.dismissViewControllerAnimated(true, completion: nil) } }
|
執行結果
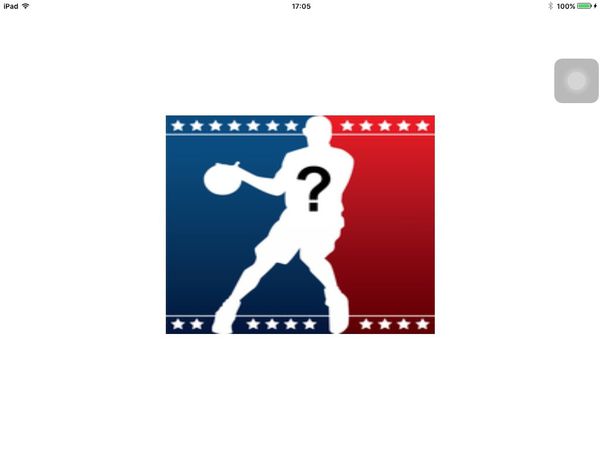
點擊並選擇照片
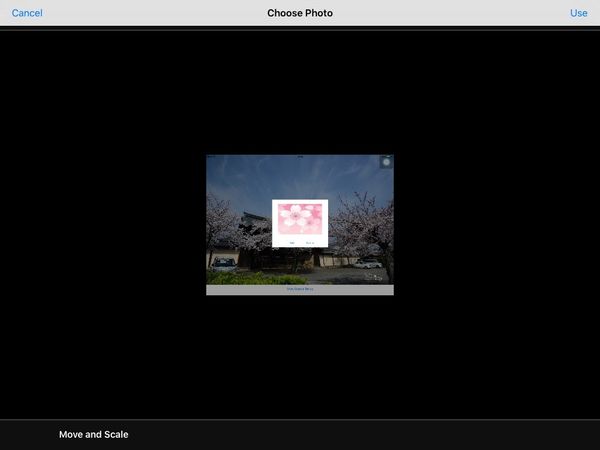
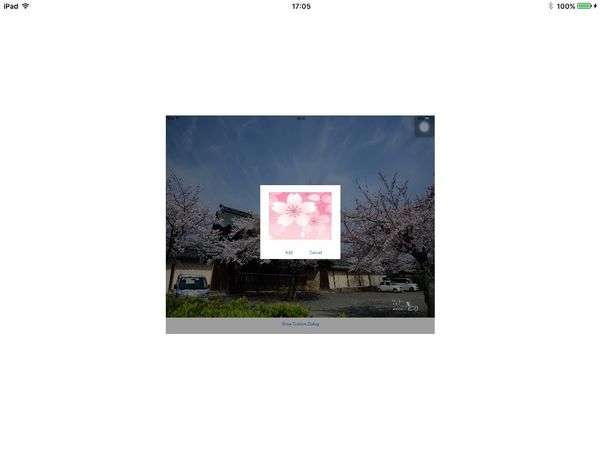
Source Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| import UIKit
class ViewController: UIViewController {
@IBOutlet weak var imageField: UIImageView! override func viewDidLoad() { super.viewDidLoad()
let tapGestureRecognizer = UITapGestureRecognizer(target:self, action:#selector(ViewController.imageClick(_:))) imageField.userInteractionEnabled = true imageField.addGestureRecognizer(tapGestureRecognizer) } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() }
internal func imageClick(img: AnyObject) {
if UIImagePickerController.isSourceTypeAvailable(.PhotoLibrary){ let picker = CustomImagePicker() picker.delegate = self picker.sourceType = UIImagePickerControllerSourceType.PhotoLibrary picker.allowsEditing = true; self.presentViewController(picker, animated: true, completion: { () -> Void in }) } else { print("Can't read the album!") } } }
extension ViewController : UIImagePickerControllerDelegate {
func imagePickerController(picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [String : AnyObject]) {
let image = info[UIImagePickerControllerOriginalImage] as! UIImage imageField.image = image; picker.dismissViewControllerAnimated(true, completion: nil) } }
extension ViewController : UINavigationControllerDelegate { }
|